Fail - Generate a V4 failure
Usage
|
result = Fail ( ) |
|
result = Fail ( errorMsg ) |
|
result = Fail ( tagarg ) |
Parameters
|
result |
This module does not return a result. |
|
errorMsg |
The failure error message. |
|
tagarg |
One of the tagged arguments below. |
Tagged Results
|
Message? |
Returns the last errorMsg. If there is no prior message then the module fails. |
|
Reset? |
Clears out any prior errorMsg. |
Description
This module simply fails. If an error is given then that text becomes the failure reason, otherwise a generic reason is provided. This module is similar to the NOp module (with an argument of 0) except that Fail can specify a failure reason.
FinCoupon - Calculations for Financial Coupons
Usage
|
result = FinCoupon ( restag? arg arg ... ) |
Parameters
|
result |
The result of the module- a number or date depending on restag. |
|
restag |
One of the tags listed below indicating the desired result. |
|
arg |
Tagged arguments as described below. |
Tagged Arguments
|
Basis::npt |
The type of count to use. The format is days-in-month/days-in-year where 0 (default)=30/360, 1=actual/actual, 2=actual/360, 3=actual/365. |
|
Frequency::npt |
The frequency of the coupon, 1=annual, 2=semiannual, 4=quarterly (mandatory). |
|
Maturity::dpt |
The maturity date (mandatory). |
|
Settlement::dpt |
The settlement date (mandatory). |
Tagged Results
|
DateAS? |
Return the next coupon date after settlement |
|
DateBS? |
Return then coupon date before settlement |
|
DaysBS? |
Return the from the coupon date before settlement to the settlement date. |
|
DaysNC? |
Calculate the number of days from settlement to the next coupon date. |
|
DaysS? |
The number of days in the coupon period that contains the settlement date. |
|
NumSM? |
Number of coupon periods between settlement and maturity. |
Description
The FinCoupon module performs the various calculations as called for by the Tagged Results.
Examples
|
FinCoupon(DaysBS? Settlement::D:960125 Maturity::D:971115 Frequency::2)
FinCoupon(DaysS? Settlement::D:960125 Maturity::D:971115 Frequency::2)
FinCoupon(DateAS? Settlement::D:960125 Maturity::D:971115 Frequency::2)
FinCoupon(NumSM? Settlement::D:960125 Maturity::D:971115 Frequency::2)
FinCoupon(DateBS? Settlement::D:960125 Maturity::D:971115 Frequency::2)
|
FinCvtDec - Converts a Fractional Dollar To Decimal
Usage
|
dollars = FinCvtDec ( fracdol frac ) |
Parameters
|
dollars |
The dollar amount in decimal format |
|
fracdol |
A dollar amount expressed as a fractional amount. |
|
frac |
The fractional units (denominator), usually 4, 8, or 16. |
Description
Converts a fractional representation of dollars to standard decimal form. In the fractional form, the decimal value reflects, typically, an eighth or sixteenth. For example 1.04 with a base of 16 is really 1 + 4/16 = 1.25.
Examples
|
FinCvtDec( 1.08 16 ) |
returns 1.50 (8/16 = .5) |
|
FinCvtDec( 1.1 4 ) |
returns 1.25 (1/4 = .25) |
FinCvtFrac - Converts Decimal Dollar to Fractional Equivalent
Usage
|
fracdol = FinCvtFrac ( dollars frac ) |
Parameters
|
fracdol |
The dollar amount in fractional format. |
|
dollars |
A dollar amount in decimal (normal) format. |
|
frac |
The fraction denominator, usually 4, 8, or 16. |
Description
This module is the inverse of FinCvtDec. It converts from standard decimal form to a fractional form.
Examples
|
FinCvtFrac( 1.50 16 ) |
returns 1.08 (.50 = 8/16) |
|
FinCvtFrac( 1.125 8 ) |
returns 1.1 (.125 = 1/8) |
FinDays360 - Returns Days Between Two Dates on a 12/30 Calendar
Usage
|
days = FinDays360 ( date1 date2 ) |
Parameters
|
days |
The number of days between the two dates |
|
date1 |
The starting date |
|
date2 |
The ending date. |
Description
This module calculates the number of days, on a 12 month/30 day calendar, between two dates. In this calendar format each month has exactly 30 days and there are exactly 360 days in each year.
Examples
|
FinDays360(Date:960101 Date:970101) |
returns 360 |
|
FinDays360(Date:970201 Date:970301) |
returns 30 |
|
FinDays360(Date:960201 Date:960101) |
returns 0 (second is less than first) |
FinDep - Calculates Various Depreciation Schedules
Usage
|
depamt = FinDep ( arg arg ... ) |
Parameters
|
depamt |
The calculated depreciation amount. |
|
arg |
Tagged arguments listed below. |
Tagged Arguments
|
Cost::npt |
The initial cost. |
|
Factor::npt |
The depreciation factor (if not specified then 2). |
|
Life::npt |
The number of periods in the life of the asset. |
|
Partial::npt |
The number of months in the partial starting year (if not specified then 12). |
|
Period::npt |
The period to calculate the depreciation. |
|
Salvalge::npt |
The salvage value (if not specified then 0). |
Tagged Results
|
DDB? |
Use Double-Declining-Balance method. |
|
FDB? |
Use the Fixed-Declining-Balance method. |
|
SLN? |
Use Straight-LiNe method. |
|
SYD? |
Use the Sum-of-Years-Depreciation method. |
Description
This module is used to calculate depreciation amounts or schedules in a variety of ways as determined by its tagged arguments. The following sections describe the various depreciation methods.
Double Declining Balance
This method calculates depreciation at an accelerated rate meaning that more is depreciated at the beginning of the life than at the end. The formula used to calculate is
. For the last period the depreciation equals the remaining value (Cost-Salvage-Sum of prior years depreciation).
Fixed Declining Balance
Straight Line Depreciation
This is the simple straight-line depreciation method where the depreciation amount is constant throughout the life of the asset. The formula for calculating, assuming a full first year is
. When there is a partial first year then the formula for that year is
. The formula for the remaining periods is
. and the final period is
.
Sum of Years Depreciation
This method calculates depreciation via the sum-of-years method given by the formula-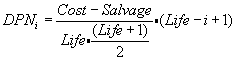
Examples
|
FinDep(SLN? Cost::100 Life::10) |
Num:10 |
|
FinDep(DDB? Cost::100 Life::10 Period::2) |
Num:16 |
|
FinDep(DDB? Cost::100 Life::10 Period::1) |
Num:20 |
FinFVSched - Calculates Future Value Based on List of Varying Rates
Usage
|
fv = FinFVSched ( principal list ) |
Parameters
|
fv |
The calculated future value. |
|
principal |
Initial starting principal |
|
list |
A list of periodic interest rates. It is assumed that the periods are the same for each rate. |
Description
FinFVSched calculates the future value of the initial principal after applying a list of compounded interest rates.
Examples
|
FinFVSched(1 (.09 .11 .1)) |
Num:1.331 |
FinIntRate - Calculates Interest Rates
Usage
|
rate = FinIntRate ( arg arg ... ) |
Parameters
|
rate |
The calculated interest rate as determined by Tagged Result. |
|
arg |
A tagged argument, see below. |
Tagged Arguments
|
Compounds::npt |
The number of compounds. If none specified then continuous compounding is assumed. |
|
Effective::npt |
The effective interest rate. |
|
Inflation::npt |
The inflation rate (default is 0.0) |
|
Nominal::npt |
The nominal interest rate. |
|
TaxRate::npt |
The effective tax rate (default is 0.00) |
Tagged Results
|
Effective? |
Returns the effective interest rate. Required tags are Nominal. Optional tags are Compounds, Inflation, and TaxRate. |
|
Nominal? |
Returns the nominal interest rate. Required tags are Effective and Compounds. |
Description
This module determines various interest rates based on the tagged result and tagged arguments. The examples below show the types of calculations that can be performed with this module.
Examples
|
FinIntRate(Effective? Nominal::.08 Compounds::12) |
Num:0.0829995 |
FinIRR - Internal Rate of Return on List of Cash Flows
Usage
|
rate = FinIRR ( list ) |
|
rate = FinIRR ( tagarg ) |
Parameters
|
rate |
Calculated rate of return. |
|
list |
A list of cash flows. |
|
tagarg |
One or more tagged arguments below. |
Tagged Arguments
|
Enum::list |
A list of points to enumerate through. |
|
First::num |
The first point to be used in the calculation (typically a negative cash outflow). |
|
Values::isct |
An intersection to be evaluated for each point in the Enum::list. The resulting list is used as the numeric list for the calculation. |
Description
This module returns, through an iterative calculation, the internal rate of return for a list of period cash flows. Payments are represented by negative values, income as positive values.
Examples
|
FinIRR( (-100 15 25 10 10 25 22) ) |
Num:0.0187956 |
|
FinIRR( (15 25 10 10 25 22) First::-100 ) |
same as above |
FinNPV - Net Present Value on List of Cash Flows
Usage
|
npv = FinNPV ( rate cashflow ) |
Parameters
|
npv |
The calculated net-present-value |
|
rate |
The discount rate for one period of the investment. |
|
cashflow |
A list of cash flows (payments are negative, income is positive) |
Description
FinNPV calculates the net present value of an investment based on a list of cash flows and discount rate. The formula used is 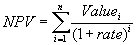
Examples
|
FinNPV( .012 (-40 20 15 -10 5 18) ) |
Num:6.4856 |
FinSecDisc - Discount Rate for a Security
Usage
|
value = FinSecDisc ( tagarg tagarg ... ) |
Parameters
|
value |
The discount rate of the security. |
|
tagarg |
One of the tagged arguments below. |
Tagged Arguments
|
Basis::npt |
The calendar basis for the calculations (0 for 30/360, 1 for actual/actual, 2 for actual/360, and 3 for actual/365). The Default is 0. |
|
Maturity::dpt |
The maturity date. |
|
Price::npt |
The initial purchase price of the security |
|
Redemption::npt |
The redemption value of the security. |
|
Settlement::dpt |
The settlement date of the security. |
Description
FinSecDisc returns the discount rate of a security.
Examples
FinSecDur - Duration of a Security
Usage
|
value = FinSecDur ( tagres tagarg tagarg ... ) |
Parameters
|
value |
The calculated duration as specified by tagres. |
|
tagres |
One of the tagged results listed below. |
|
tagarg |
One or more of the tagged arguments below depending on tagres. |
Tagged Arguments
|
Basis::npt |
The calendar basis for the calculations (0 for 30/360, 1 for actual/actual, 2 for actual/360, and 3 for actual/365). The Default is 0. |
|
Coupon::npt |
The annual coupon rate. |
|
First::dpt |
The date of first coupon payment (defaults to first coupon date after settlement). |
|
Frequency::npt |
The number of coupon payments per year: 1, 2, or 4. |
|
Issue::dpt |
The issue date (defaults to Settlement). |
|
Maturity::dpt |
The maturity date. |
|
Settlement::dpt |
The settlement date of the security. |
|
Yield::npt |
The annual yield. |
Tagged Results
|
Macaulay? |
Calculates the Macaulay duration for a security. |
|
Modified? |
Calculates the modified Macaulay duration for a security. |
Description
This module returns the Macaulay duration (or modified) for a security. It can be used as a measure of a bond price's response to any changes in the yield.
Examples
FinSecInt - Accrued Interest (or Rate) for a Security
Usage
|
value = FinSecInt ( tagres tagarg tagarg ... ) |
Parameters
|
value |
The calculated interest as specified by tagres. |
|
tagres |
One of the tagged results listed below. |
|
tagarg |
One or more of the tagged arguments below depending on tagres |
Tagged Arguments
|
Basis::npt |
The calendar basis for the calculations (0 for 30/360, 1 for actual/actual, 2 for actual/360, and 3 for actual/365). The default is 0. |
|
Coupon::npt |
The annual coupon rate. |
|
First::dpt |
The date of the first interest. |
|
Frequency::npt |
The number of coupon payments per year. |
|
Investment::npt |
The amount invested in the security. |
|
Issue::dpt |
The security's issue date. |
|
Maturity::dpt |
The maturity date. |
|
Par::npt |
The securities par value, default is $100. |
|
Rate::npt |
The annual coupon rate. |
|
Redemption::npt |
The redemption value, or amount to be received at maturity. |
|
Settlement::dpt |
The settlement date of the security. |
Tagged Results
|
Maturity? |
Returns the accrued interest of a security paying interest at maturity. The required tag are Issue, Rate, and Settlement. Optional tags are Basis and Par. |
|
Periodic? |
Calculates the accrued interest of a security paying periodic interest. Required tags are Basis, Coupon, First, Frequency, Issue, and Settlement. Optional are Par and Basis. |
|
Rate? |
Returns the interest rate for a fully invested security. Required tags are Investment, Maturity, Redemption, and Settlement. |
Description
This module performs various interest calculations for a security.
Examples
FinSecPrice - Price of a Security
Usage
|
value = FinSecPrice ( tagres tagarg tagarg ... ) |
Parameters
|
value |
The calculated price as specified by tagres. |
|
tagres |
One of the tagged results listed below. |
|
tagarg |
One or more of the tagged arguments below depending on tagres. |
Tagged Arguments
|
Basis::npt |
The calendar basis for the calculations (0 for 30/360, 1 for actual/actual, 2 for actual/360, and 3 for actual/365). The default is 0. |
|
First::dpt |
The date of the first interest. |
|
Frequency::npt |
The number of coupon payments per year. |
|
Issue::dpt |
The security's issue date. |
|
Last::dpt |
The security's last coupon date. |
|
Maturity::dpt |
The maturity date. |
|
Rate::npt |
The annual coupon rate. |
|
Redemption::npt |
The redemption value, or amount to be received at maturity. |
|
Settlement::dpt |
The settlement date of the security. |
|
Yield::npt |
The annual yield. |
Tagged Results
|
Discount? |
Return the price of a discounted security. Required tags are Discount, Maturity, Redemption, and Settlement. Basis is optional. |
|
Maturity? |
Return the price of a security paying interest at maturity. Required tags are Issue, Maturity, Rate, Settlement, and Yield. Basis is optional. |
|
OddFirst? |
Calculate the price of a security with an odd first period. Required tags are First, Frequency, Issue, Maturity, Rate, Settlement, and Yield. Basis is optional. |
|
OddLast? |
Calculate the price of a security with an odd last period. Required tags are Frequency, Issue, Last, Maturity, Rate, Redemption, Settlement, and Yield. Basis is optional. |
|
Periodic? |
Returns the price of a security paying periodic interest. Required tags are Frequency, Maturity, Rate, Redemption, Settlement, and Yield. Basis is optional. |
Description
Examples
FinSecRcvd - Amount Received for a Security
Usage
|
value = FinSecRcvd ( tagarg tagarg ... ) |
Parameters
|
value |
The amount received at maturity for a fully invested security. |
|
tagarg |
One of the tagged arguments below. Note that all of the tags below must be given with the exception of Basis. |
Tagged Arguments
|
Basis::npt |
The calendar basis for the calculations (0 for 30/360, 1 for actual/actual, 2 for actual/360, and 3 for actual/365). The default is 0. |
|
Discount::npt |
The discount rate |
|
Investment::npt |
The amount invested in the security. |
|
Maturity::dpt |
The maturity date. |
|
Settlement::dpt |
The settlement date of the security. |
Description
Examples
FinSecYield - Calculates the Yield of a Security
Usage
|
value = FinSecYield ( tagres tagarg tagarg ... ) |
Parameters
|
value |
The calculated yield as specified by tagres. |
|
tagres |
One of the tagged results listed below. |
|
tagarg |
One or more of the tagged arguments below depending on tagres |
Tagged Arguments
|
Basis::npt |
The calendar basis for the calculations (0 for 30/360, 1 for actual/actual, 2 for actual/360, and 3 for actual/365). The default is 0. |
|
First::dpt |
The date of the first interest. |
|
Frequency::npt |
The number of coupon payments per year. |
|
Issue::dpt |
The security's issue date. |
|
Last::dpt |
The security's last coupon date. |
|
Maturity::dpt |
he maturity date. |
|
Price::npt |
The price paid for the security. |
|
Rate::npt |
The annual coupon rate. |
|
Redemption::npt |
The redemption value, or amount to be received at maturity. |
|
Settlement::dpt |
The settlement date of the security. |
Tagged Results
|
Discount? |
Return the annual yield of a discounted security. Required tags are Maturity, Price, Redemption, and Settlement. Basis is optional. |
|
Maturity? |
Return the annual yield of a security paying interest at maturity. Required tags are Issue, Maturity, Price, Rate, and Settlement. Basis is optional |
|
OddFirst? |
Calculate the annual yield of a security with an odd first period. Required tags are First, Frequency, Issue, Maturity, Price, Rate, Redemption, and Settlement. Basis is optional. |
|
OddLast? |
Calculate the annual yield of a security with an odd last period. Required tags are Frequency, Issue, Last, Maturity, Price, Rate, Redemption, and Settlement. Basis is optional. |
|
Periodic? |
Returns the yield of a security paying periodic interest. Required tags are Frequency, Maturity, Price, Rate, Redemption, and Settlement. Basis is optional. |
Description
Examples
FinTBill - T bill Calculations
Usage
|
result = FinTBill ( tagres arg arg ... ) |
Parameters
|
result |
Result of calculation as determined by tagres. |
|
tagres |
One of the tagged results points listed below indicating type of calculation. |
|
arg |
One or more of the tagged arguments described below. |
Tagged Arguments
|
Discount::npt |
The discount rate. |
|
Maturity::dpt |
The maturity date. |
|
Price::npt |
The price per $100. |
|
Settlement::dpt |
The settlement date. |
Tagged Results
|
BondEqYield? |
Calculate the bond-equivalent yield. |
|
Price? |
Calculate the price per $100 face value. |
|
Yield? |
Calculate the yield. |
Description
The FinTBill module performs various calculations commonly used with treasury bills
Examples
|
FinTBill(BondEqYield? Settlement::D:960115 Maturity::D:961230 Discount::.093) |
Num:0.103518 |
|
FinTBill(Price? Settlement::D:960115 Maturity::D:961230 Discount::.093) |
Num:91.0875 |
FinTVM - Performs Various Time Value of Money Calculations
Usage
|
result = FinTVM ( restag arg arg ... ) |
Parameters
|
result |
The result of the module- a number or date depending on restag. |
|
restag |
One of the tags listed below indicating the desired result. |
|
arg |
Tagged arguments as described below. |
Tagged Arguments
|
EPeriod::dpt |
The ending period for cumulative calculations (for IPmt? and PPmt? calculations). |
|
FV::npt |
The future value, default is 0. |
|
Nth::npt |
The particular period (for IPmt? and PPmt? calculations) |
|
Periods::npt |
The number of periods. |
|
Pmt::npt |
The periodic payment. |
|
PV::npt |
The present value. |
|
Rate::npt |
The periodic interest rate. |
|
SPeriod::dpt |
The start period for cumulative calculations (for IPmt? and PPmt?). |
Tagged Results
|
FV? |
Calculate the future value. |
|
IPmt? |
Calculate the interest portion of a payment. |
|
Periods? |
Calculate the number of periods. |
|
Pmt? |
Calculate the periodic payment. |
|
PPmt? |
Calculate the principal portion of a payment. |
|
PV? |
Calculate the present value. |
|
Rate? |
Calculate the periodic interest rate. |
Description
The FinTVM module, in its basic form, takes four of the five tagged arguments: FV, Periods, Pmt, PV, and Rate and calculates the fifth. When calculating IPmt and PPmt, either the Nth argument or both the EPeriod and SPeriod arguments must be specified. The equations used in these calculations are-
Examples
The first example below returns the present value (PV?). The second returns the future value (FV?). The third example returns the sum of all principal payments which should equal the present value of 1400. The next two examples return the cumulative interest payments for periods 13 through 24 and for just period 1. The last two return cumulative principal payments for the same periods.
|
FinTVM( Rate::.01 Pmt::-100 FV::0 Periods::12 PV? )
FinTVM( FV? Rate::.01 Pmt::-100 PV::1000 Periods::12 )
Sum(EnumCL(Int:1..12 @FinTVM(PPmt? Rate::.01 PV::1400 Periods::12 Nth::Int*)))
FinTVM(IPMT? Rate::.0075 PV::125000 Periods::360 SPeriod::13 EPeriod::24 )
FinTVM(IPMT? Rate::.0075 PV::125000 Periods::360 SPeriod::1 EPeriod::1 )
FinTVM(PPmt? Rate::.0075 PV::125000 Periods::360 SPeriod::13 EPeriod::24 )
FinTVM(PPmt? Rate::.0075 PV::125000 Periods::360 SPeriod::1 EPeriod::360 )
|
Flatten - Flattens Multiple Points into a Single Point
Usage
|
truept = Flatten ( tag dim1 dim2 ... ) |
|
flatpt = Flatten ( point1 point2 ... ) |
Parameters
|
truept |
Logical:True is returned when this module is used to define a flatten pattern. |
|
flatpt |
The resulting flattened point derived from the argument points. |
|
pointn |
The points to be flattened. |
Tagged Arguments
|
Target::dim |
This argument defines the target dimension when defining a flatten pattern. If given then all other arguments must be dimension points. |
Description
This module is used to combine multiple points into a single (flattened) point. It is to be used in conjunction with the Tally module when grouping with multiple By points results in slow performance or errors.
Flatten is normally called once to define a flatten pattern. This is done by calling the module with two or more dimension points. An optional Target dimension may be specified. If no target is given then the target dimension becomes Dim:UBlob.
Once a flatten pattern is defined then any subsequent bindings are checked for points within the pattern. If a match is found (all points in the pattern occur within the binding), the points are removed from the binding and the flattened point is inserted. This occurs with all bindings including those at the end of a Tally operation.
When bindings of the form [nidpoint point1 point2]=value are constructed V4 will automatically construct another binding of the form [nidpoint dim1.. dim2..=[nidpoint Flatten(dim1* dim2*)].
The net-net of all this is that the Flatten module can be called once to define a pattern and that no other actions or code changes need be done to a V4 analysis.
Examples
Suppose a Tally such as the one below fails because there are too many combinations of dim.attr2 and dim.attr3 values (and that dim.attr2 results in points on Dim:Dim2 and dim.attr3 results in points on Dim:Dim3).
|
Tally(dim.. (Sum:dim.attr1 By::(dim.attr2 dim.attr3) Bind::sum23))
|
The addition of the Flatten below creates a pattern that is detected when new bindings are made. Additionally, a binding is created (shown below) that automatically links the Tally results to the new Flatten module.
|
Flatten(Dim:Dim2 Dim:Dim3)
[sum23 Dim2.. Dim3..] = [sum23 Flatten(Dim2* Dim3*)]
|
Format - Format a Point for Display
Usage
|
respt = Format ( argument tagarg ... ) |
Parameters
|
respt |
The formatted/altered number, may be alpha or numeric depending on tagargs. |
|
argument |
A point to be formatted or any V4 point to be converted to a string (or other V4 point). |
|
tagarg |
One or more of the tagged arguments below. |
Tagged Arguments
|
Center::width |
Centers result in string width spaces. |
|
Dim::dimension |
Format the subsequent arguments according to the parameters for dimension as declared in the last SSDim module call. Multiple dimensions may be included in a single Format call to specify any manner of embedded formating. See example below. |
|
EQ::num |
Skip the next formatting if current numeric argument is not equal to num. |
|
Fill::char |
Use the character char as the fill character(s) with the Left, Right, & Center tagged arguments. The default is to fill with spaces. |
|
Fill::string |
Use this string as a suffix with the Maximum tag when the string exceeds the specified maximum length. |
|
GE::num |
Skip the next formatting if current numeric argument is not greater than or equal to num. |
|
GT::num |
Skip the next formatting if current numeric argument is not greater than num. |
|
LE::num |
Skip the next formatting if current numeric argument is not less or equal to num. |
|
Left::width |
Left justifies result in string width spaces. |
|
LT::num |
Skip the next formatting if current numeric argument is not less than num. |
|
Mask::mask |
Converts the argument according to the specifications of the mask. V4 supports masks for telephone numbers, numerics, dates, date-times, and months. See the table below for more details. A mask may be given in three parts for numeric formating. The parts are delimited with a semicolon. The first part is for formatting positive numbers. The second is for negative numbers and the third for zero. If only two formats are specified then zero is formatted as a positive number. A mask may be given in two parts for dates. The first part is for real dates, the second for 'no date'. When a format is given for a zero or 'no date' then the actual format string is returned (i.e. it is not considered a format mask but a string to use). |
|
Mask::cmask |
If a mask string begins with the percent symbol ('%') then it is assumed to be a C-language format expression. |
|
Maximum::len |
Truncate the string to len characters if necessary. If a Fill string is given then append that string to the end of the truncated string such that the resulting string is exactly len characters in length. |
|
NE::num |
Skip the next formatting if current numeric argument is not equal to num. |
|
Num::repeat |
Repeat the current string repeat times (for example to create any number of spaces for indenting, or a list of dashes for a table of contents. |
|
RDB::rdbtype |
Formats the first argument to be a valid SQL value for the specified rdbtype. The supported database types are MIDAS, MSAccess, , and Oracle. See the Dimension attribute RDBNumeric to force formatting of internal values. If the point belongs to an alpha dimension with the CaseSensitive attribute and the rdbtype is MYSQL then the keyword 'binary' is inserted to force case-sensitive matching. |
|
RDBId::rdbtype |
Formats an SQL column identifier according to the syntax required by the rdbtype engine. For example, mySQL identifiers are enclosed within back-tics (`xxx). |
|
RDBw::rdbtype |
Similar to the RDB tag except that it is intended for formatting values to be included in an SQL WHERE clause. The RDB tag is only defined for single points, the RDBw tag is defined for single points, and point references that incorporate greater than, less than, not equal, etc. V4 formats only the single value in the point reference. |
|
Right::width |
Right justifies result in string width spaces. |
|
Round::tens |
Rounds the numeric argument to the specified power of ten. |
|
Round::decimals |
Rounds the numeric argument to the specified number of decimal places. decimals must be between 0 and 8. |
|
Round::npt |
Round number to specified precision. |
|
Table::name |
Creates a string formatted according to the table name. The first argument point is the owner point of the table. |
|
Value::string |
Return the string as the formatted result. |
Tagged Results
|
CSV? |
Format the point as a valid CSV value. |
|
Point? |
Returns a string corresponding to the dimension & point value of the first argument. |
|
Range? |
Converts the point specification to a text range (see examples below). The specific wording for this option is found in the V4 error message file (V4ErrorMsgTable.v4i) under the entries- FormatRngxxx. For example the wording for greater-equal would be under then entry FormatRngGE, for equal it is FormatRngEQ, etc. |
|
UTC? |
Formats the first argument, which must be a UDate, DateTime, or Calendar, according to the Javascript toUTCstring() function. |
|
XML? |
Formats the first argument as a valid argument for remote procedure calls. |
Description
The Format module formats V4 points in a variety of ways. The formatting is always applied to the first argument. One or more of the tagged arguments is specified to perform the required formatting. The arguments Left, Right, and Center are used to position the result within a given width. The comparison tags are used for conditional formatting. If the condition is met then the next tagged argument is used for formatting. If the condition is not met then the next argument is skipped and the following one is used.
A value normally takes as many positions as is necessary to fully represent the value. The tags mentioned above may be used to position a value within a fixed column width. If a width (via Left, Right, or Center is specified before a Mask argument AND the resulting value will not fit within a given width then the module fails. If a width is specified after the mask then the formatted value is truncated to fit.
This module operates on various types of points. The Left, Right, and Center tags require that the first argument be a string. V4 will convert non-string points to Dim:Alpha if necessary. If this occurs the result is returned as a point on Dim:Alpha.
Table 10 - Numeric Formatting Characters
Pattern |
Meaning |
# |
Defines a numeric position. |
. |
Defines the decimal position or the thousands delimiter as specified by the current country setting (see Locale). |
0 |
Defines leading zero-filled positions before the decimal point and number of places after the decimal point. |
, |
Delimits the thousands positions or the decimal point. The meaning depends on the current country setting for V4 (see Locale). Some countries use this as to separate every three digits, other countries use it for marking the decimal point. |
_ |
Mark the position of the thousands' delimiter. The default for Country:USA is to then insert commas every three places. Other countries may insert spaces or periods. |
! |
Mark the position of the decimal point. The actual character used depends on the current country setting. The default for Country:USA is the period character. |
( |
Enclose negative numbers within parentheses (default is to use leading minus sign). |
x |
Format number as hexadecimal. |
o |
Format number as octal. |
b |
Format number as binary. |
th |
Display the number as an ordinal (e.g. 1st, 2nd, 3rd, 4th, etc.). |
w |
Display the number as written words (e.g. 123 as One hundred twenty three). |
[color] |
A color may be enclosed in brackets. This may be used to color positive or negative numbers (see example below). |
Date & Time formatting
Table 11 - Date-Time Formatting Characters
Pattern |
Meaning |
mm |
Display the month as a two digit space filled number. |
0m |
Display the month as a two digit zero filled number. |
yy |
Display the year as a two digit zero filled number. |
yyyy |
Display the year as a four digit century and year. |
dd |
Display the day of the month as a two digit space filled number. |
0d |
Display the day as a two digit zero filled number. |
h |
Display the hour (0 - 23). |
H |
Display the hour (12 - 11). |
0h |
Display the hour zero filled. |
a |
Display 'am' or 'pm'. |
A |
Display 'AM' or 'PM'. |
mmm |
Display the month as upper/lower case three letter month abbreviation. |
MMM |
Display the month as upper case three letter abbreviation. |
mmmm |
Display the month as upper/lower full month name. |
MMMM |
Display the month as all upper case. |
n |
Display the minutes (m is used for months!). |
0n |
Dislay the minutes as z zero filled number |
jjj |
Display the day in the year (1 through 365) as space filled number. |
0jj |
Display the day as a zero filled number. |
s |
Display the seconds. |
f |
Display the fractional seconds. |
0s |
Display the seconds zero filled. |
www |
Display the day of the week as a three letter abbreviation. |
wwww |
Display the day of the week. |
WWW |
Display the day of the week as all upper case. |
z |
Output the time zone as hhmm (hours/minutes) off of GMT. |
p |
Display the period (1-4). |
q |
Display the quarter (1-4). |
k |
Displays the week number (1-52). |
all other |
All other characters are displayed as is allowing for many possible date displays. If a sub-portion of the mask is enclosed in double quotes then the characters between those quotes will be taken literally, not as format characters. The backslash may be used as an escape character within the nested quoted string. The format mask can be comprised of up to three semi-colon delimited sections. The first part corresponds to a fully defined telephone number, the second to a number without an area code, the third for formatting an empty phone number. |
Phone Number formatting
Table 12 - Phone Number Formatting
Pattern |
Meaning |
a |
Displays the area code |
c |
Displays the country code |
n |
Displays the number portion. Each digit of the number is represented by a single occurance of the 'n' character. Other characters can be used to delimit groups. |
Examples
|
Format(12.456 Round::2) |
results in Num:12.46 |
|
Format(123456 Mask::"#,###") |
results in "123,456" |
|
Format(1234 Round::100) |
results in 12 |
|
Format(12345 mask::"#,###" center::12 Fill::"*") |
results in "***12,345***". |
|
Format(-1234.3 Mask::"#,###.00;[RED](#,###.00)") |
results in (1,234.30) |
|
Format(UDate:010101 Mask::"mmmm d, yyyy") |
results in "January 1, 2001" |
|
Format(UDate:#0 Mask::"mmmm d, yyyy") |
results in an empty string |
|
Format(UDate:#0 Mask::"mmmm d, yyyy;none") |
results in "none" |
|
Format(UWeek:200522 Mask::"yyyy\" Week #\"k") |
results in "2005 Week #22" |
|
Format(1234 EQ::0 Value::"zip" Mask::"#,###") |
results in "1,234" (or "zip" if first argument is 0) |
|
Format(Int* Mask::"#,###;#,###;zip") |
also returns "zip" if Int* is 0 |
|
Format(Int:>20 Range?) |
results in "more than 20" |
|
Format(UMonth* Mask::"mmm")/UMonth:=UYear:{current} |
returns the list ("Jan" "Feb" ... "Dec") |
|
Format(V4(ConnectTime?) Mask::"0n:0s.ffff") |
results in (for example) "23:42.8320" |
|
Format("xo" Num::5) |
xoxoxoxoxo |
|
Format(teleNum:6109899905 Mask::"c-aaa-nnn.nnnn") |
results in "1-610-989.9905" |
|
Format(teleNum:none Mask::"c-aaa-nnn.nnnn;1-610-nnn-nnnn;*no phone*") |
results in "*no phone*" |
Example 28 - Multiple Embedded Formatting Within a String
|
Dim Red,IBlue Alpha
SSDim(Dim:Red TextColor::Red Dim:IBlue TextColor::Blue Style::Italic)
EchoS(Format("now is " Dim:Red "the time " "for all " Dim:IBlue "good men"))
results in "now is the time for all good men"
|
FTP - Put / Get Files via FTP
Usage
Parameters
|
result |
The result of the module which varies according to the arguments |
|
tagarg |
One or more tagged arguments described below |
Tagged Arguments
|
As::file |
The target file name for the get or put. |
|
Get::list |
A list of filenames to be gotten. |
|
Get::file |
The name of the file to be gotten. |
|
Host::name |
The name or IP address of the host FTP server. |
|
Local::directory |
The name of the local directory to connect to for the get/put operation. |
|
Password::string |
The login password for the target FTP server. |
|
Put::file |
The name of the file to be sent. |
|
Put::list |
A list of files to be sent. |
|
Remote::directory |
The name of the remote directory to connect to. |
|
Result::type |
The format of the returned result. If type is Bytes then the total number of bytes sent/received is returned, if Files (the default) then the total number of files is returned, if Listthen a list of files is returned. |
|
Trace::logical |
If TRUE then turn on tracing, FALSE (default) to disable tracing. |
|
User::name |
The user name for the target FTP server. |
Tagged Results
|
Data? |
The data to be sent/received is binary. |
|
Text? |
The data to be sent/received is text. |
Description
This module send/receives files to/from a remote host using the FTP file transfer protocol.
Examples
|
FTP(Host::"remHost" Remote::"/user/holding" Local::"temp/" Get::"afile.v4" As::"moo.tmp" User::"vic" Password::"secret") |
Gets file afile.v4 as moo.tmp |
|
FTP(Host::"192.196.222.332" Remote::"/user/holding" Put::(Alpha*)/OSFile("*.ini" ListOf?) User::"vic" Password::Secure(Value::"ftp.password") Result::list)) |
Sends a list of files |